Colored text in bsui#
Section Topic
Many of the example code blocks in the sections showing code developed at BMM show functions and tools used at BMM which are not standard parts of IPython or Bluesky. This section shows code listings for those things.
- Lesson
Develop convenient tools for applying color and style to text written to the bsui terminal.
- Beamline
- Link to source code
- Section author
Bruce Ravel (bravel@bnl.gov)
Colorful terminal text#
BMM users do a lot at the command line. It is helpful to have tools for emphasizing text printed to the screen in various ways. Happily, IPython provides good tools for applying ASCII colors and accents to terminal text. The following is some syntactic sugar making those tools easier to use.
There are several examples of the colored text shortcuts in the status method shown in the Linkam code listing.
1 from IPython.utils.coloransi import TermColors as color
2
3 def colored(text, tint='white', attrs=[]):
4 '''
5 A simple wrapper around IPython's interface to TermColors
6 '''
7 tint = tint.lower()
8 if 'dark' in tint:
9 tint = 'Dark' + tint[4:].capitalize()
10 elif 'light' in tint:
11 tint = 'Light' + tint[5:].capitalize()
12 elif 'blink' in tint:
13 tint = 'Blink' + tint[5:].capitalize()
14 elif 'no' in tint:
15 tint = 'Normal'
16 else:
17 tint = tint.capitalize()
18 return '{0}{1}{2}'.format(getattr(color, tint), text, color.Normal)
19
20 def error_msg(text):
21 '''Red text'''
22 return colored(text, 'lightred')
23 def warning_msg(text):
24 '''Yellow text'''
25 return colored(text, 'yellow')
26 def go_msg(text):
27 '''Green text'''
28 return colored(text, 'lightgreen')
29 def url_msg(text):
30 '''Text without decoration, reserved for URL decoration...'''
31 return colored(text, 'normal')
32 def bold_msg(text):
33 '''Bright white text'''
34 return colored(text, 'white')
35 def verbosebold_msg(text):
36 '''Bright cyan text'''
37 return colored(text, 'lightcyan')
38 def list_msg(text):
39 '''Cyan text'''
40 return colored(text, 'cyan')
41 def disconnected_msg(text):
42 '''Purple text'''
43 return colored(text, 'purple')
44 def info_msg(text):
45 '''Brown text'''
46 return colored(text, 'brown')
47 def whisper(text):
48 '''Light gray text'''
49 return colored(text, 'darkgray')
This, then, associates different colors and accents on the text to meaningful words. “Error” means bright red text. “Go” means bright, green text, and so on.
Examples:
print(go_msg('Scan finished successfully!'))
print(error_msg('Unable to do the thing...'))
print(whisper('don\'t forget to open the shutter'))
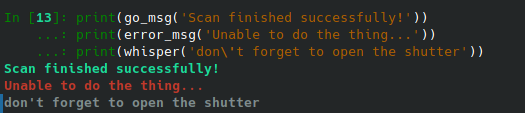
Fig. 8 Examples of colored text#
Boxed blocks of text#
Additional emphasis can be placed on a block of text by putting it in
a box with a title line at the top. This uses the colored()
function shown above to color the characters making up the walls of
the box.
The Linkam status display is an example of this
in use, with some of the interior text being displayed using the
colored()
function described above.
1import ansiwrap
2
3def boxedtext(title, text, tint, width=75):
4 '''
5 Put text in a lovely unicode block element box. The top
6 of the box will contain a title. The box elements will
7 be colored.
8 '''
9 remainder = width - 2 - len(title)
10 ul = u'\u2554' # u'\u250C'
11 ur = u'\u2557' # u'\u2510'
12 ll = u'\u255A' # u'\u2514'
13 lr = u'\u255D' # u'\u2518'
14 bar = u'\u2550' # u'\u2500'
15 strut = u'\u2551' # u'\u2502'
16 template = '%-' + str(width) + 's'
17
18 print('')
19 print(colored(''.join([ul, bar*3, ' ', title, ' ', bar*remainder, ur]), tint))
20 for line in text.split('\n'):
21 lne = line.rstrip()
22 add = ' '*(width-ansiwrap.ansilen(lne))
23 print(' '.join([colored(strut, tint), lne, add, colored(strut, tint)]))
24 print(colored(''.join([ll, bar*(width+3), lr]), tint))
Example:
text = '''Double, double toil and trouble;
Fire burn and caldron bubble.
Fillet of a fenny snake,
In the caldron boil and bake;
Eye of newt and toe of frog,
Wool of bat and tongue of dog,
Adder's fork and blind-worm's sting,
Lizard's leg and howlet's wing,
For a charm of powerful trouble,
Like a hell-broth boil and bubble.
'''
boxedtext('Song of the Witches', text, 'brown', width=45)
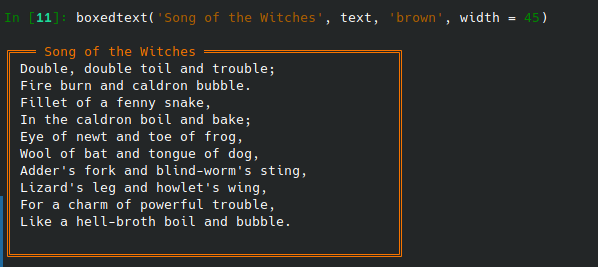
Fig. 9 Example of boxed text with a brown border#