Additional features of the Linkam class#
Linkam status#
Because the status_code
is such a obscure way of presenting status
information, I made a small display showing the most salient features
of the controller.
linkam.status()
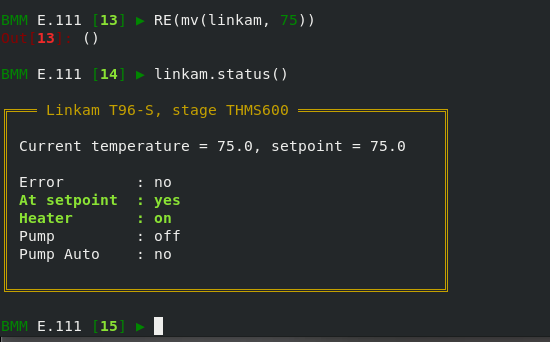
Fig. 4 A quick-n-dirty status display for the Linkam.#
This display decodes the bits in linkam.status_code
. The temperature
and set point are given. At the top of the box, are the model numbers of the
controller and stage.
Note that the status()
method makes use of convenience tools
explained in Colored text in bsui.
String attributes#
If you do this:
linkam.model_array.get()
the returned value is
array([84, 57, 54, 45, 83, 0], dtype=uint8)
an array of integers. Huh?
The key is to recognize that the integers are ASCII code points for the letters of the alphabet. This translates to “T96-S”, the model of the controller.
Here is the translation code:
1 def arr2word(self, lst):
2 word = ''
3 for l in lst[:-1]:
4 word += chr(l)
5 return word
6
7 @property
8 def model(self):
9 return self.arr2word(self.model_array.get())
Now model
is a property of the class which returns the string
tranlation of the model_array
.
linkam.model
returns ‘T96-S’
One of these properties exists for each of the attributes returning an integer array. See Code Listing, lines 74-95.
Turning the Linkam on and off#
Finally, there is a way to turn the heater stage on and off, with versions convenient for the bsui command line and versions better suited for use in a proper bluesky experimental plan.
1 def on(self):
2 self.startheat.put(1)
3
4 def off(self):
5 self.startheat.put(0)
6
7 def on_plan(self):
8 return(yield from mv(self.startheat, 1))
9
10 def off_plan(self):
11 return(yield from mv(self.startheat, 0))
At the bsui command line:
linkam.on()
linkam.off()
In an experimental plan:
yield from linkam.on_plan()
yield from linkam.off_plan()